Class 11 Chapter 9: List
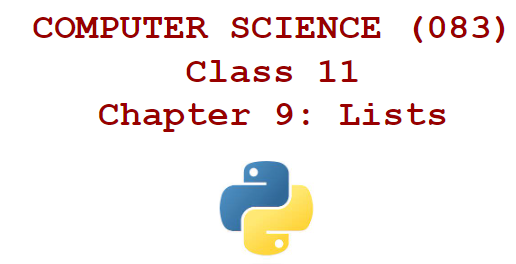
- Write a program to increment elements of a list by 5.
a=[10,20,30,40,50]
print("actual list",a)
for i in range(len(a)):
a[i]+=5
print("after increment",a)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
actual list [10, 20, 30, 40, 50]
after increment [15, 25, 35, 45, 55]
2. Write a menu driven program to perform any four list operations.
a=[10,20,30,40,50]
print("actual list",a)
b=int(input("""
Menu of options
1. append
2. pop
3. insert
4. length
"""))
if b==1:
var1=int(input("Enter the element to append"))
a.append(var1)
print("updated list",a)
elif b==2:
a.pop()
print("updated list",a)
elif b==3:
var2=int(input("Enter the index number"))
var3=int(input("ENter the element to insert"))
a.insert(var2,var3)
print("updated list",a)
elif b==4:
print("length of list",len(a))
else:
print("please enter a valid option")
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
actual list [10, 20, 30, 40, 50]
Menu of options
1. append
2. pop
3. insert
4. length
1
Enter the element to append60
updated list [10, 20, 30, 40, 50, 60]
3. Write a program to calculate average marks of the student where n is entered by the user.
a=[]
n=int(input("Enter max number"))
for i in range(n):
b=int(input("Enter the marks"))
a.append(b)
print("avg marks",sum(a)/len(a))
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter max number4
Enter the marks40
Enter the marks50
Enter the marks60
Enter the marks70
avg marks 55.0
4. Write a program to check if a number is present in the list or not. If the number is present, return the position of the number. Print an appropriate message if the number is not present.
a = eval(input("Enter list: "))
b = int(input("Enter number to search: "))
if b in a:
print(b, "found at index", a.index(b))
else :
print(b, "not found in list")
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter list: [1,2,3,6,8,9]
Enter number to search: 10
10 not found in list
5. Write a program to find the number of times an element occurs in the list.
a = eval(input("Enter list: "))
b = int(input("Enter number to find the occurence: "))
if b in a:
print(b, "occurs ", a.count(b),"times")
else :
print(b, "not found in list")
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter list: [1,1,2,3,4,5,5,6,7]
Enter number to find the occurrence: 5
5 occurs 2 times
6. Write a program to read a list of n integers(positive as well as negative). Create two new lists, one having all positive numbers and other having all negative numbers from the given list. Print all the three lists.
a = eval(input("Enter list: "))
b=[]
c=[]
for i in a:
if i>0:
b.append(i)
else:
c.append(i)
print("all the element",a)
print("list with positive elements",b)
print("list with negative elements",c)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter list: [1,2,3,-2,-5,23,-45]
all the element [1, 2, 3, -2, -5, 23, -45]
list with positive elements [1, 2, 3, 23]
list with negative elements [-2, -5, -45]
7. Write a program to return the largest element from the list of numbers.
a = eval(input("Enter list: "))
print("largest value is:",max(a))
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter list: [1,2,3,45,5,6]
largest value is: 45
8. Write a program to return the second largest number from the list of numbers.
a = eval(input("Enter list: "))
a.sort()
print("second largest value is:",a[-2])
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter list: [1,2,3,45,5,6]
second largest value is: 6
9. Write a program to read a list on n integers and find their median.
lst = eval(input("Enter a list :-"))
lst.sort()
length = len(lst)
if length % 2 == 0 :
med = (lst[length//2-1]+lst[(length//2)])/2
else :
med = lst [ (length // 2)]
print("Median :-",med)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[1,2,3,45,5,6]
Median :- 4.0
10. Write a program to read a list of elements. Modify this list so that it does not contain any duplicate elements ,i.e., all elements occurring multiple times in the list should appear only once.
lst = eval(input("Enter a list :-"))
lst1=[]
for i in lst:
if i not in lst1:
lst1.append(i)
print(“list without duplicate elements”,lst1)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[1,1,2,3,4,5,5]
list without duplicate elements[1, 2, 3, 4, 5]
11. Write a program to read a list of elements. Input an element from the user that has to be inserted in the list. Also input the position to insert the element at the desired position.
lst = eval(input("Enter a list :-"))
a=int(input("ENter the element"))
b=int(input("Enter the index position"))
lst.insert(b,a)
print("modified list",lst)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[1,1,2,3,4,5,5]
ENter the element6
Enter the index position2
modified list [1, 1, 6, 2, 3, 4, 5, 5]
12. Write a program to read elements of a list.
a) The program should ask for the position of the element to be deleted and delete the element at the desired position from the list.
b) The program should ask for the value of the element to be deleted from the list and delete the same from the list.
a)
lst = eval(input("Enter a list :-"))
a=int(input("ENter the position of element to be deleted"))
lst.pop(a)
print("updated list",lst)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[1,1,2,3,4,5,5]
ENter the position of element to be deleted6
updated list [1, 1, 2, 3, 4, 5]
b)
lst = eval(input("Enter a list :-"))
a=int(input("ENter the element to be deleted"))
lst.remove(a)
print("updated list",lst)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[10,20,30,40,50]
ENter the element to be deleted40
updated list [10, 20, 30, 50]
13. Write a program to read elements and reverse the elements without creating a new list.
lst = eval(input("Enter a list :-"))
lst.reverse()
print("reverse list",lst)
Output:
= RESTART: C:/Users/rainb/OneDrive/Documents/Desktop/1.py =
Enter a list :-[10,20,30,40,50]
reverse list [50, 40, 30, 20, 10]